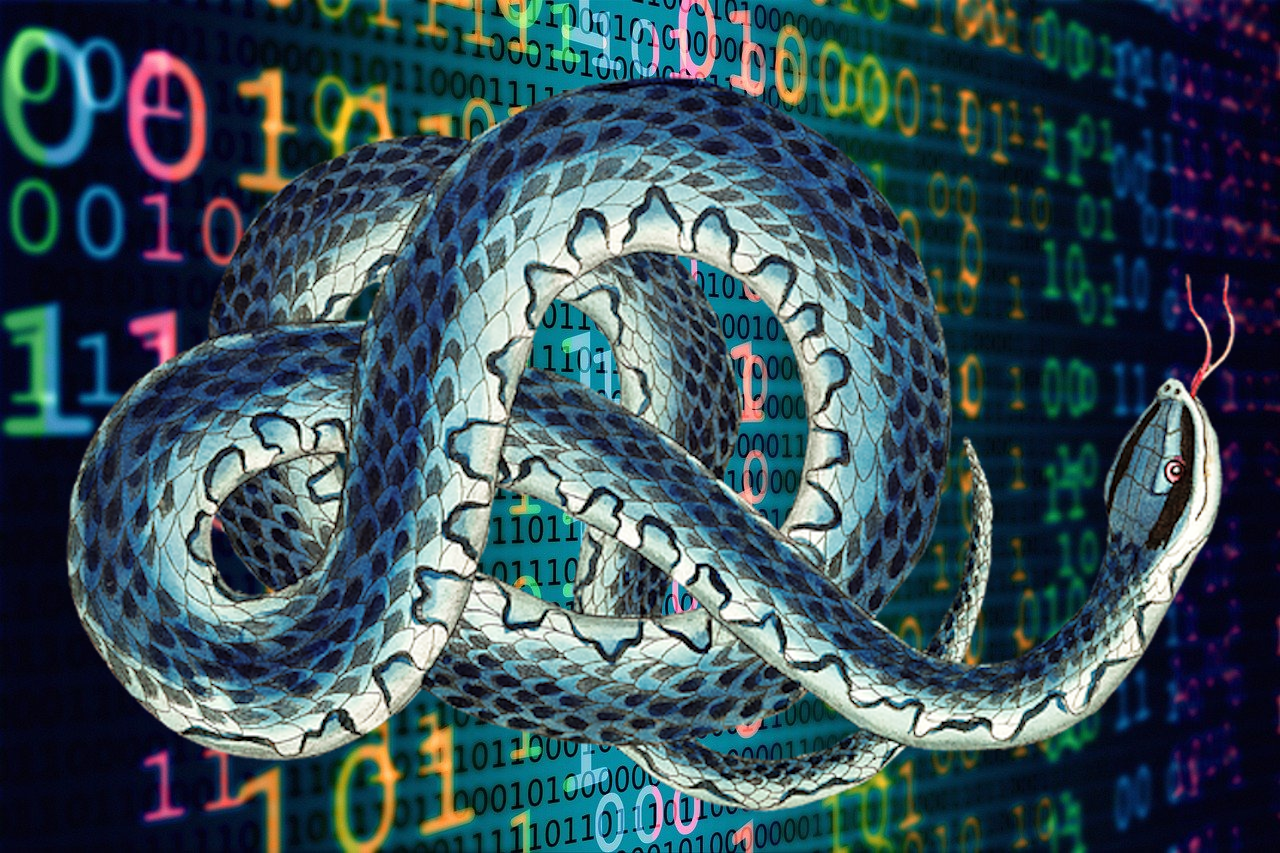
Recommended Programming Courses
What Is My WiFi IP Address? Find With Python
How to find whats your WiFi IP address using Python programming language. This tutorial will show you how to get your IP address using Python.
There are many ways to find your IP address. You can use the command line, a web browser, or a Python script. In this tutorial, we will show you how to find your IP address using Python.
Python is a powerful programming language that is widely used for web development, data analysis, and machine learning. It is also a great tool for network programming. In this tutorial, we will show you how to use Python to find your IP address.
There are many ways to find your IP address using Python. You can use the socket module, the requests module, or the ipaddress module. In this tutorial, we will show you how to use the socket module to find your IP address.
The socket module is a built-in module in Python that provides a low-level interface for network programming. It allows you to create sockets, send and receive data over the network, and find your IP address.
Here is a simple Python script that will find your IP address using the socket module:
import socket
def get_ip_address():
try:
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
s.connect(("8.8.8.8", 80))
ip_address = s.getsockname()[0]
except Exception as e:
print(f"An error occurred: {e}")
ip_address = None
finally:
s.close()
return ip_address
Python Code Explanation
The get_ip_address function creates a UDP socket using the socket.socket function. It then connects the socket to the Google Public DNS server (
You can use this piece of code to figure out what is the IP address of your WiFi network. Please note that this method is not 100% accurate. It may return the IP address of your network interface, but it may not be the IP address that is visible to the outside world. You can find what is your external IP address using findWhatIsMyIP.com.
Other Languages to Find Your WiFi IP Address
Besides Python, there are several other programming languages you can use to find your WiFi IP address. Here are a few examples:
1. JavaScript
Using JavaScript, you can find your IP address by making a request to an external service. Here is an example using the fetch API:
fetch('https://api.ipify.org?format=json')
.then(response => response.json())
.then(data => console.log(data.ip))
.catch(error => console.error('Error:', error));
2. Bash
In a Unix-based system, you can use a simple Bash command to find your IP address:
ip addr show | grep 'inet ' | awk '{print $2}' | cut -d/ -f1
3. C#
Using C#, you can find your IP address with the following code:
using System;
using System.Net;
class Program
{
static void Main()
{
string hostName = Dns.GetHostName();
IPAddress[] addresses = Dns.GetHostAddresses(hostName);
foreach (IPAddress address in addresses)
{
if (address.AddressFamily == System.Net.Sockets.AddressFamily.InterNetwork)
{
Console.WriteLine(address.ToString());
}
}
}
}
4. Go
Using Go, you can find your IP address with the following code:
package main
import (
"fmt"
"net"
)
func main() {
addrs, err := net.InterfaceAddrs()
if err != nil {
fmt.Println(err)
return
}
for _, addr := range addrs {
if ipnet, ok := addr.(*net.IPNet); ok && !ipnet.IP.IsLoopback() {
if ipnet.IP.To4() != nil {
fmt.Println(ipnet.IP.String())
}
}
}
}
Which Language is the Fastest?
The speed of finding your IP address depends on various factors, including the efficiency of the language's networking libraries and the overhead of the runtime environment. Generally, lower-level languages like C and Go tend to be faster due to their minimal runtime overhead. However, for most practical purposes, the difference in speed is negligible, and you should choose the language you are most comfortable with or the one that best fits your project's requirements.
TCP/IP Socket Programming in C#