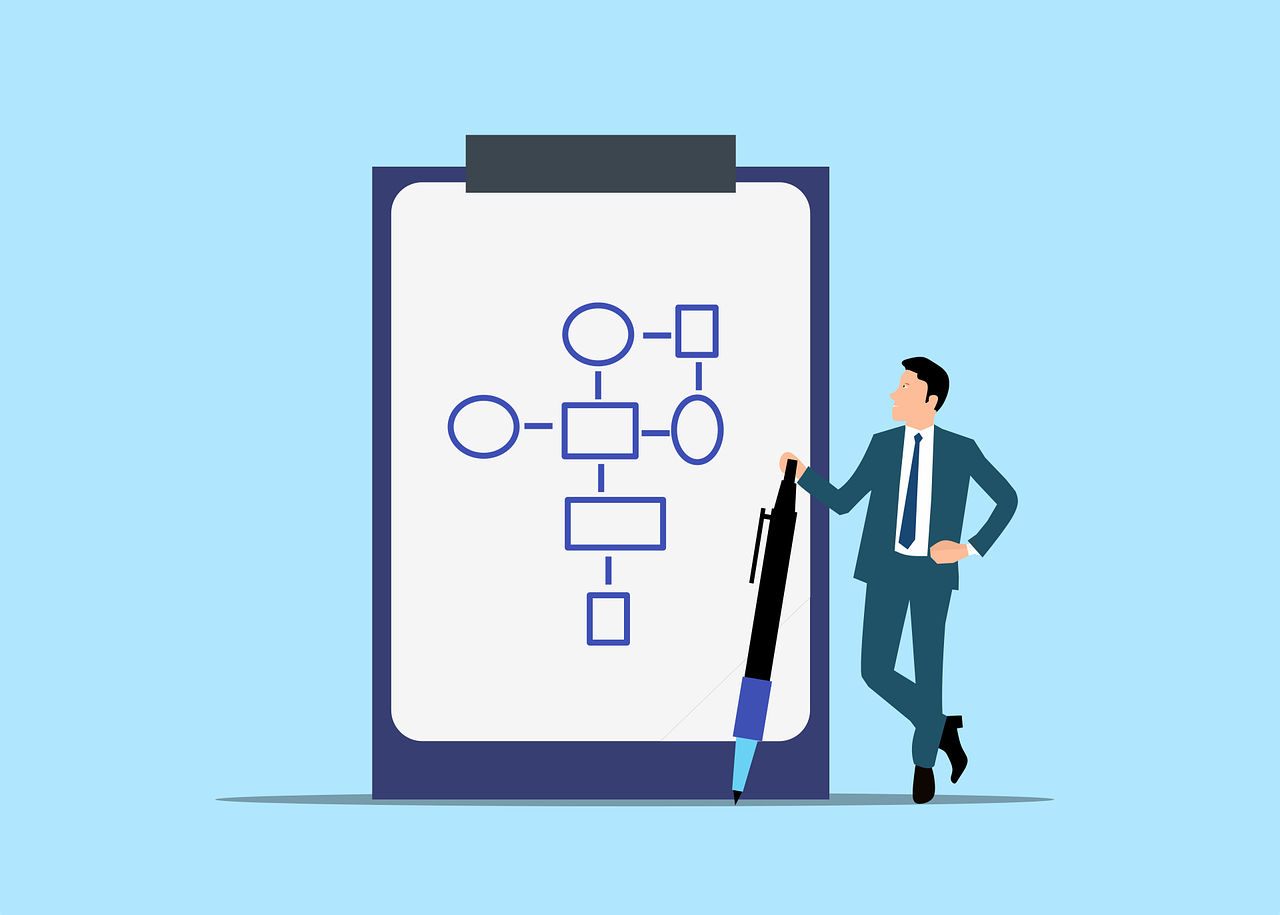
Recommended Programming Courses
What is Regular Expression and Use with IP Address
A regular expression, a.k.a regex (plural regexes) a sequence of characters that define a search pattern. Usually such patterns are used by string searching algorithms for "find" or "find and replace" operations on strings, or for input validation.
Regular expressions are based on a formal language that is used to represent a regular grammar. The subject is known ad "Theory of Automata".Examples of Regular Expressions
- IP Address Validation Regex for IPV4
^(?:(?:25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)\.){3}(?:25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)$
- IP Address Validation Regex for IPV6
^([0-9a-fA-F]{1,4}:){7}([0-9a-fA-F]{1,4}|:)$
- Email Validation:
^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$
- IP Address Validation:
^(?:[0-9]{1,3}\.){3}[0-9]{1,3}$
- URL Validation:
^(https?|ftp)://[^\s/$.?#].[^\s]*$
- Phone Number Validation:
^\+?(\d[\d-. ]+)?(\([\d-. ]+\))?[\d-. ]+\d$
- Postal Code Validation (US):
^\d{5}(-\d{4})?$
Regular expressions are used in search engines, search and replace dialogs of word processors and text editors, in text processing utilities such as sed and AWK and in lexical analysis.
Pros and Cons of Regexes
Pros
- Powerful
- Flexible
- Fast
- Portable
- Widely supported
Cons
- Complex
- Hard to read
- Hard to write
- Hard to debug
- Hard to maintain
Regex Engines
There are many regex engines available, some of the popular ones are:
- Perl Compatible Regular Expressions (PCRE)
- Java Regular Expressions
- Python Regular Expressions
- JavaScript Regular Expressions
- PHP Regular Expressions
- GNU Regular Expressions
- POSIX Regular Expressions
Regex Tools
There are many tools available to test and debug regular expressions. Some of the popular ones are:
- Regex101
- RegExr
- RegExPal
- RegExr
- RegExr
- RegExr
- RegExr
Regex in Programming Languages
Regular expressions are supported in many programming languages. Programming languages with first class support for regular expressions let you define variables of regex type. Other programming languages support the use of regexes through libraries. Some of the popular ones are:
- Python
- JavaScript
- Java
- PHP
- Ruby
- C#
- Perl
Example: Using Regex in Python
import re
# Sample text
text = "The quick brown fox jumps over the lazy dog."
# Regex pattern to find all words
pattern = r'\b\w+\b'
# Find all matches
matches = re.findall(pattern, text)
print(matches)
Example use of Regex in JavaScript
// Sample text
const text = "The quick brown fox jumps over the lazy dog.";
// Regex pattern to find all words
const pattern = /\b\w+\b/g;
// Find all matches
const matches = text.match(pattern);
console.log(matches);
Using Regex to find IP Addresses
Regular expressions provide a handy tool to search for IP addresses through large amounts of data. Here is an example of using regex to find IP addresses in a block of text:
Example: Using Regex to Find IP Addresses in Python
import re
# Sample text containing IP addresses
text = "Here are some IP addresses: 192.168.1.1, 10.0.0.1, and 172.16.0.1."
# Regex pattern to find IPv4 addresses
pattern = r'\b(?:[0-9]{1,3}\.){3}[0-9]{1,3}\b'
# Find all matches
matches = re.findall(pattern, text)
print(matches)
Example: Using Regex to Find IP Addresses in JavaScript
// Sample text containing IP addresses
const text = "Here are some IP addresses: 192.168.1.1, 10.0.0.1, and 172.16.0.1.";
// Regex pattern to find IPv4 addresses
const pattern = /\b(?:[0-9]{1,3}\.){3}[0-9]{1,3}\b/g;
// Find all matches
const matches = text.match(pattern);
console.log(matches);
These examples demonstrate how you can use regular expressions to search for and extract IP addresses from text in both Python and JavaScript. The regex pattern used here matches typical IPv4 addresses.