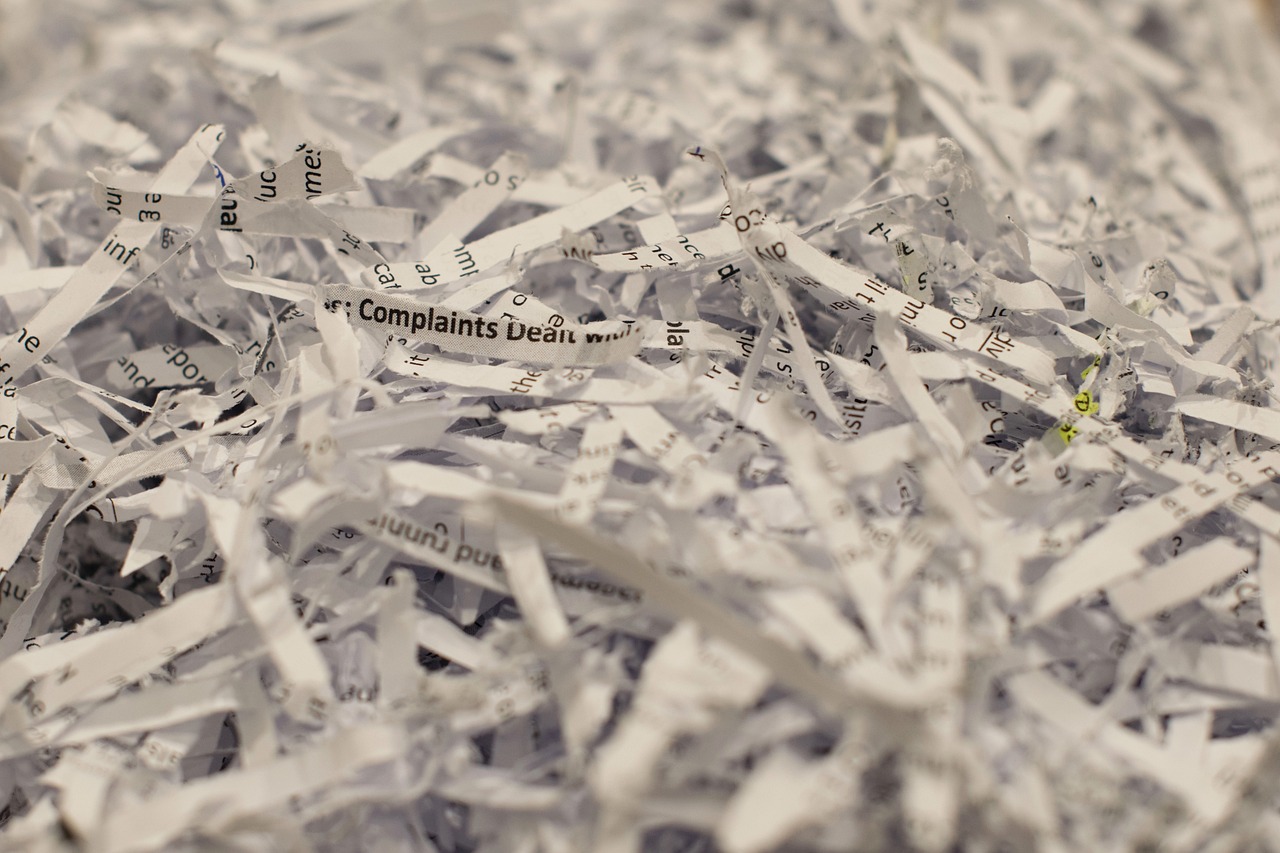
Understanding File Shredding
Imagine you finished reading a secret letter from a lover. If you throw the letter in the bin, it might be discovered by a blackmailer(cyber criminal) in the future. Tearing up a letter in two won't be enough since anybody could use some solution tape and common sense to assemble your secrets. That's what normal file/folder deletion does — the data can often be recovered using special tools.
But Why Shred?
File shredding is a method used to permanently delete files from a computer system, making them irrecoverable. While this approach ensures that sensitive information does not fall into the wrong hands, it comes with its own set of risks and caveats.
NIST CSF and File Shredding
The National Institute of Standards and Technology's Cybersecurity Framework (NIST CSF) emphasizes the importance of protecting information through processes like data deletion and file shredding. File shredding fits into the Protect function of NIST CSF, which aims to safeguard sensitive data by ensuring it cannot be recovered once deleted. Adopting proper shredding practices aligns with the CSF's objective of preventing unauthorized access to sensitive information.
The Importance of Proper File Disposal
File shredding addresses this issue by not just deleting but overwriting the data multiple times. It is crucial in fields like cybersecurity, data protection, and personal privacy management. Just like a secretive spy, it's about covering your tracks entirely.
Risks Involved
There's a story of a company that learned the hard way: improperly shredded files led to a massive security leak. Proper shredding practices could have prevented this mishap. But beware, if done incorrectly, you might lose data that you later realize is crucial.
In Conclusion
File shredding is a powerful tool for managing sensitive information but requires knowledge and awareness to wield without endangering data unnecessarily. Consider it your shield in the digital realm, defended by sound practices.
How to Do File Shredding in Python
Python provides several ways to effectively shred files, ensuring that the data is not recoverable. Below is a simple method to overwrite the contents of a file before deletion:
import os
# Function to shred a file
def shred_file(file_path, passes=3):
"""
Overwrite the file with random data, then delete it.
:param file_path: Path to the file to be deleted.
:param passes: Number of times to overwrite the file.
"""
try:
with open(file_path, 'ba+', buffering=0) as delfile:
length = delfile.tell()
for _ in range(passes):
delfile.seek(0)
delfile.write(os.urandom(length))
delfile.seek(0)
delfile.truncate()
os.remove(file_path)
print(f"{file_path} has been shredded.")
except Exception as e:
print(f"Error shredding file: {e}")
# Example usage:
# shred_file('path/to/file.txt')
How to Do File Shredding in C#
C# offers robust libraries that can be used to implement file shredding. Below, we demonstrate how you can create a simple command-line application to securely delete files. We'll separate the file shredding logic into its own class for clarity and maintainability.
using System;
using System.IO;
// Class responsible for shredding files
public class FileShredder
{
public void ShredFile(string filePath, int passes = 3)
{
// Validate the file exists
if (!File.Exists(filePath))
{
Console.WriteLine($"File {filePath} does not exist.");
return;
}
// Obtain the file length
long length = new FileInfo(filePath).Length;
try
{
// Overwrite file contents
using (FileStream stream = new FileStream(filePath, FileMode.Open))
{
Random random = new Random();
byte[] randomData = new byte[length];
for (int i = 0; i < passes; i++)
{
random.NextBytes(randomData);
stream.Seek(0, SeekOrigin.Begin);
stream.Write(randomData, 0, randomData.Length);
}
}
// Truncate the file
using (FileStream stream = new FileStream(filePath, FileMode.Truncate))
{
stream.SetLength(0);
}
// Delete the file
File.Delete(filePath);
Console.WriteLine($"{filePath} has been shredded.");
}
catch (Exception ex)
{
Console.WriteLine($"Error shredding file: {ex.Message}");
}
}
}
// Entry point for the command-line application
class Program
{
static void Main(string[] args)
{
if (args.Length < 1)
{
Console.WriteLine("Usage: FileShredder.exe [passes]");
return;
}
string filePath = args[0];
int passes = args.Length > 1 ? int.Parse(args[1]) : 3;
FileShredder shredder = new FileShredder();
shredder.ShredFile(filePath, passes);
}
}
How to Do File Shredding in PowerShell
PowerShell, a task automation and configuration management framework from Microsoft, also offers methods to securely delete files by overwriting them. Here's a simple way to shred a file using PowerShell:
# Function to securely delete a file by overwriting it
function Shred-File {
param (
[Parameter(Mandatory=$true)]
[string]$FilePath,
[int]$Passes = 3
)
if (Test-Path $FilePath) {
try {
# Get file length
$file = Get-Item $FilePath
$length = $file.Length
# Create buffer with random data
$random = New-Object System.Random
$buffer = New-Object byte[] $length
[System.IO.FileStream]$fs = [System.IO.File]::Open($FilePath, [System.IO.FileMode]::Open)
for ($i = 0; $i -lt $Passes; $i++) {
$random.NextBytes($buffer)
$fs.Seek(0, "Begin")
$fs.Write($buffer, 0, $buffer.Length)
}
$fs.Close()
# Delete the file
Remove-Item $FilePath
Write-Host "$FilePath has been shredded."
}
catch {
Write-Host "Error shredding file: $_"
}
} else {
Write-Host "File $FilePath does not exist."
}
}
# Example usage:
# Shred-File -FilePath 'C:\path\to\file.txt'
Popular File Shredding Software Solutions
- Eraser: Free, open-source Windows tool for secure data removal.
- File Shredder: Easy-to-use, free file shredding software for Windows.
- CCleaner: Comprehensive system optimization and cleaning tool with file shredding capabilities.
- Securely File Shredder: Android app for safely deleting files.
- WipeDrive: Software for complete wiping of hard drives, sometimes used for enterprise-level data security.
Conclusion
File shredding is an essential aspect of data security and personal privacy. Properly shredding files ensures sensitive information is virtually unrecoverable, safeguarding against unauthorized data access. By understanding and implementing reliable file shredding techniques within programming languages like Python and C#, you can significantly reduce the risk of data breaches and maintain high levels of security. As technology continues to advance, ensuring data is properly managed and disposed of will remain a crucial responsibility for individuals and organizations alike.