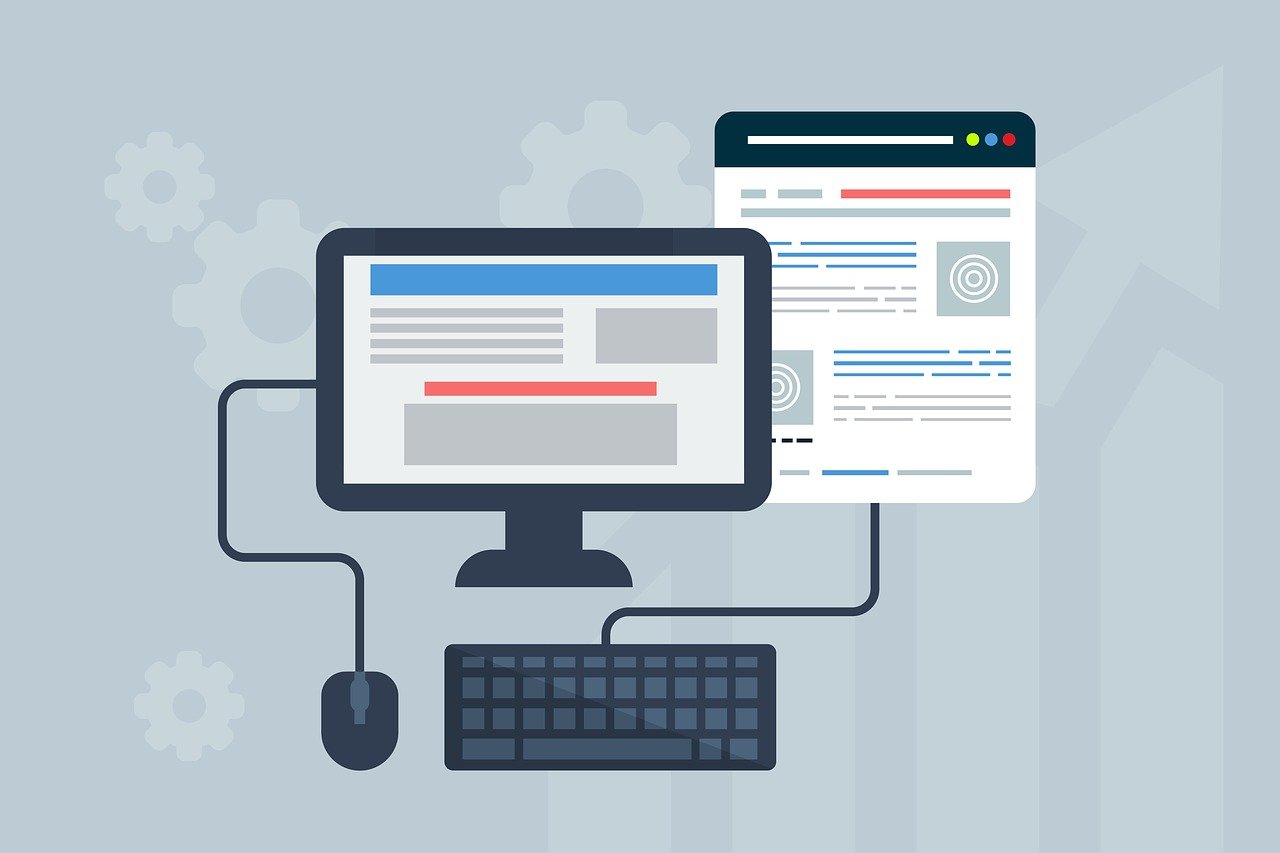
Recommended Programming Courses
What is WebSockets
WebSocket is a computer communications protocol, providing full-duplex communication channels over a single TCP connection. It is designed to be implemented in web browsers and web servers, but it can be used by any client or server application. WebSocket is distinct from HTTP but is designed to work over HTTP ports 80 and 443 as well as to support HTTP proxies and intermediaries, making it compatible with the existing web infrastructure.
Websockets pros and cons are listed below for you.
Pros of WebSockets
Websockets offer several advantages, please read the pros of web-socket listed below:
- Low Latency: WebSockets provide low-latency communication, making them ideal for real-time applications such as chat applications, online gaming, and live data feeds.
- Full-Duplex Communication: Unlike HTTP, which is request-response based, WebSockets allow for full-duplex communication, meaning both the client and server can send and receive data simultaneously.
- Reduced Overhead: WebSockets reduce the overhead of HTTP headers, leading to more efficient use of resources and bandwidth.
Cons of WebSockets
Despite their advantages, WebSockets also have some drawbacks or cons:
- Complexity: Implementing WebSockets can be more complex compared to traditional HTTP communication.
- Compatibility: Not all browsers and network intermediaries fully support WebSockets, which can lead to compatibility issues.
- Security: WebSockets can be vulnerable to certain types of attacks if not properly secured.
Security Implications
WebSockets can introduce security risks if not properly managed. Some of the security implications include:
- Cross-Site WebSocket Hijacking: Attackers can hijack WebSocket connections if proper authentication and authorization mechanisms are not in place.
- Denial of Service (DoS): WebSockets can be used to launch DoS attacks by overwhelming the server with a large number of connections.
- Data Interception: Without encryption, data transmitted over WebSockets can be intercepted by attackers.
To mitigate these risks, it is important to use secure WebSocket connections (wss://), implement proper authentication and authorization, and monitor WebSocket traffic for suspicious activity.
Use of IP Address and MAC Address
In the context of WebSockets, the IP address is used to establish the connection between the client and server. The IP address helps in routing the packets over the internet to the correct destination. The MAC address, on the other hand, is used within the local network to ensure that the data packets reach the correct device. While the MAC address is not directly used in the WebSocket protocol, it plays a crucial role in the underlying network communication that supports WebSocket connections.
Why WebSocket Client Needs to Know the IP Address of WebSocket Server
In the context of WebSockets, the IP address is crucial for establishing a direct connection between the client and the server. The IP address acts as a unique identifier for the server on the network, allowing the client to route its connection request to the correct destination. Without knowing the IP address, the client would not be able to locate the server and initiate the WebSocket handshake.
Using DNS When the IP Address is Not Known
When the IP address of the WebSocket server is not known, DNS (Domain Name System) can be used to resolve the server's domain name into an IP address. DNS acts as a directory service for the internet, translating human-readable domain names (e.g., example.com
) into machine-readable IP addresses (e.g., 192.0.2.1
). This allows the client to use a domain name to connect to the server, and the DNS service will handle the resolution process to find the corresponding IP address.
Step-by-Step DNS Resolution Process:
- Client Request: The WebSocket client attempts to connect to the server using its domain name (e.g.,
ws://example.com
). - DNS Query: The client's system sends a DNS query to a DNS resolver to find the IP address associated with the domain name.
- DNS Response: The DNS resolver looks up the domain name in its records and responds with the corresponding IP address.
- Connection Establishment: The client uses the resolved IP address to establish a WebSocket connection with the server.
By using DNS, clients can connect to servers without needing to know their IP addresses directly, making it easier to manage and update server addresses without affecting client configurations.
Programming Languages for WebSocket Programming
Several programming languages are commonly used for WebSocket programming, including:
- JavaScript: JavaScript is widely used for WebSocket programming, especially on the client side, due to its integration with web browsers.
- Node.js: Node.js, with its event-driven architecture, is well-suited for WebSocket programming, and libraries like `ws` make it easy to implement WebSocket servers and clients.
- Python: Python has several libraries, such as `websockets` and `aiohttp`, that make it easy to implement WebSocket servers and clients.
- Java: Java provides robust support for WebSocket programming through its Java API for WebSocket (JSR 356).
- C#: C# supports WebSocket programming with the `System.Net.WebSockets` namespace, making it a popular choice for .NET developers.
- SignalR: SignalR is a library for ASP.NET that simplifies the process of adding real-time web functionality to applications, allowing server-side code to push content to connected clients instantly.
- Go: Go's concurrency model and standard library support for WebSockets make it a good choice for building WebSocket servers.
- Ruby: Ruby has libraries like `faye-websocket` and `em-websocket` that facilitate WebSocket programming.
- Swift: Swift, primarily used for iOS development, has libraries like `Starscream` that facilitate WebSocket programming.
- Objective-C: Objective-C, also used for iOS development, can utilize libraries such as `SocketRocket` for WebSocket communication.
- Rust: Rust, known for its performance and safety, has libraries like `tokio-tungstenite` and `warp` that support WebSocket programming.
WebSocket in C++
C++ is not a popular choice for WebSocket development for several reasons:
- Complexity: C++ is a complex language with a steep learning curve, making it less accessible for developers compared to higher-level languages like JavaScript or Python.
- Lack of Libraries: While there are libraries available for WebSocket development in C++, they are not as mature or widely used as those in other languages, leading to less community support and documentation.
- Development Speed: Development in C++ can be slower due to the need for manual memory management and other low-level operations, which can be more efficiently handled by higher-level languages.
- Platform Dependency: C++ code can be more platform-dependent, requiring additional effort to ensure compatibility across different operating systems and environments.
WebSockets for Distributed Client/Server Applications
WebSockets are indeed suitable for writing distributed client/server applications. They enable real-time, bidirectional communication between clients and servers, which is essential for distributed systems. With WebSockets, you can build applications where multiple clients can interact with a server and with each other in real-time, such as collaborative tools, multiplayer games, and live data streaming services.
Inherent Problems of WebSockets Protocol
While WebSockets offer many benefits, they also come with inherent problems that developers need to be aware of:
- Scalability: Managing a large number of WebSocket connections can be challenging, especially for servers that need to handle thousands or millions of concurrent connections.
- Resource Consumption: WebSocket connections can consume significant server resources, including memory and CPU, which can impact the performance of other applications running on the same server.
- Network Interference: WebSocket connections can be affected by network intermediaries such as proxies and firewalls, which may block or interfere with WebSocket traffic.
- Protocol Overhead: Although WebSockets reduce the overhead of HTTP headers, they still introduce some protocol overhead that can impact performance, especially for small messages.
- Debugging and Monitoring: Debugging and monitoring WebSocket connections can be more complex compared to traditional HTTP connections, requiring specialized tools and techniques.
WebSocket Server and Client Examples
Please find sample WebSocket server and client code below FYI.Python WebSocket Server
import asyncio
import websockets
#Websockets sample code server-side(in Python)
async def echo(websocket, path):
async for message in websocket:
await websocket.send(message)
start_server = websockets.serve(echo, "localhost", 8765)
asyncio.get_event_loop().run_until_complete(start_server)
asyncio.get_event_loop().run_forever()
Node.js WebSocket Server
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8765 });
wss.on('connection', function connection(ws) {
ws.on('message', function incoming(message) {
ws.send(message);
});
});
WebSocket Client (JavaScript)
//Websocket sample code, client side(JavaScript)
const socket = new WebSocket('ws://localhost:8765');
socket.addEventListener('open', function (event) {
socket.send('Hello Server!');
});
socket.addEventListener('message', function (event) {
console.log('Message from server ', event.data);
});