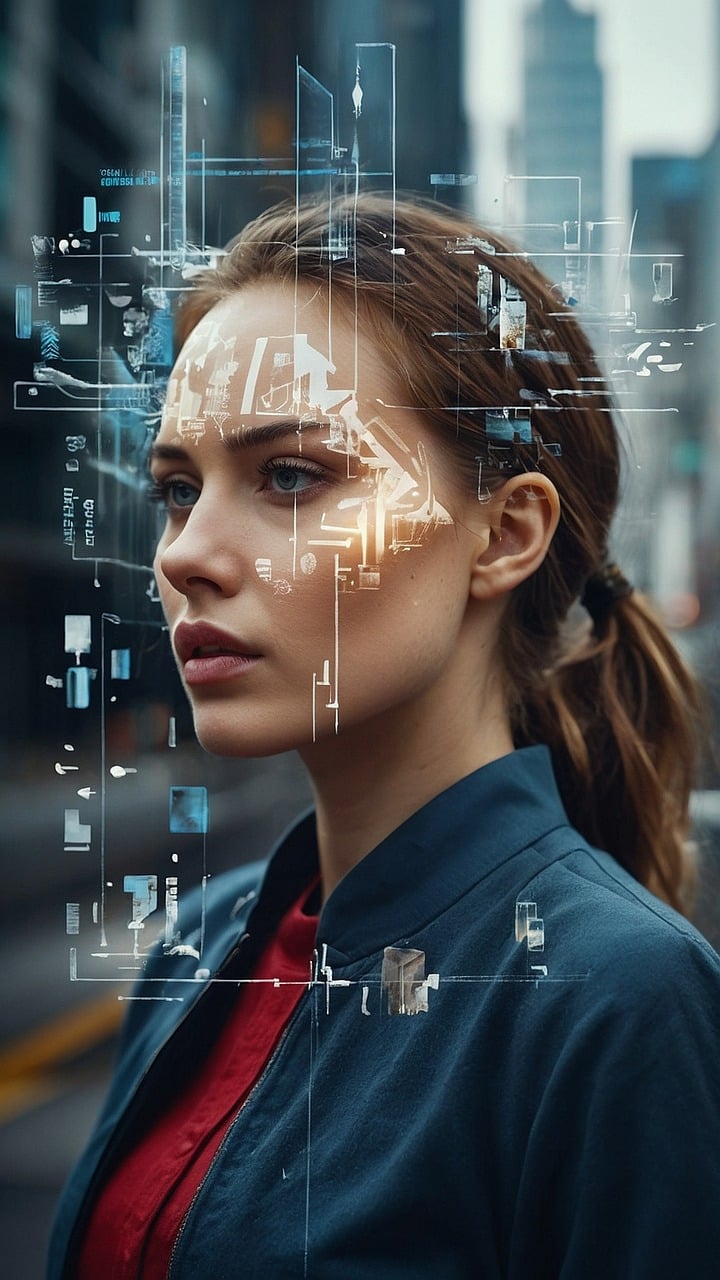
Recommended Programming Courses
What's a Port Scan with Python Code Tutorial
Imagine your computer is a house on a street filled with similar houses. Each house has a variety of doors, each leading to a different room within. Some doors you can open and enter during certain times, say for a party or a meeting, while others remain locked. Just like these houses, computers in a network have doors called 'ports'.
A network port scan is akin to walking down the street and knocking on each door of these houses to see which ones are open. Perhaps there's a friend's house you want to enter (access a service like a web page hosted on port 80 or 8080), so you'll knock on that door specifically. However, it’s crucial to respect privacy, and that's where the ethics of cybersecurity come into play, ensuring that you have permission to check which doors (or ports) are open.
In this tutorial, we are going to explore how a Python code can simulate this knocking process to identify open ports, allowing you to understand more about how networks function and how different internet services are accessed.
Uses of Port Scans in Cybersecurity
Port scans are used by various people for both good and bad reasons. Network administrators use them to assess the security and functionality of their network by identifying open ports that could be potential vulnerabilities. Professionals in cybersecurity may utilize these scans as part of a penetration test to uncover weaknesses in security defenses. However, malicious actors can also exploit port scans to find open ports and vulnerabilities to breach network defenses. Therefore, it's important to conduct port scans ethically and comply with legal regulations.
For cybercriminals, mapping a target's network is a crucial step towards launching an attack. Understanding the structure, open ports, and services running on a network enables them to identify vulnerabilities they can exploit. Port scans serve as an essential tool for this reconnaissance phase, allowing attackers to systematically check for any potential entry points into a network. Once these open ports are identified, it becomes easier for cybercriminals to plan their next steps, whether it's a data breach, network infiltration, or other malicious activities. This emphasizes the importance of being vigilant in monitoring network access and securing open ports to protect sensitive data and maintain system integrity.
Python Port Scan Sample Code
import socket
def scan_for_open_ports(findSingle=False, ports=[80, 8080]):
# This is an educational tutorial, write will not be resonsible for any damage caused by it's usage
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
try:
# doesn't even have to be reachable
s.connect(('10.255.255.255', 1))#Using a bogon IP address to find an IP address in the network
ip = s.getsockname()[0]
print(f'Starter IP: {ip}')
ip_parts = ip.split('.')
ip = '.'.join(ip_parts[:3])
ports_and_ips = {}
for i in range(0, 256):
test_ip = f"{ip}.{i}"
print(f"Testing IP: {test_ip}")
for port in ports:
if port not in ports_and_ips:
ports_and_ips[port] = []
print(f'{test_ip}:{port}')
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as sock:
sock.settimeout(0.5)
result = sock.connect_ex((test_ip, port))
if result == 0:
if findSingle:
return test_ip
print(f"===> Port {port} is open on {test_ip}")
ports_and_ips[port].append(test_ip)
else:
print(f'Port {port} is not open on {test_ip}')
except Exception as e:
print(f'Exception: {e}')
ip = '127.0.0.1'
finally:
s.close()
return ports_and_ips
This code will scan only 255 IP address in the network. You can change it to find more IP addresses but it will take more time. You can use this piece of code to find whats I P address of your home router(like XFinity, or PTCL)
How to Defend Against Port Scans
- Implement firewalls: Use firewalls to block unauthorized access to your network's ports.
- Close unnecessary ports: Regularly check and close ports not in use to reduce the number of potentially vulnerable access points.
- Use Intrusion Detection Systems (IDS): Deploy IDS to monitor network traffic and detect any unauthorized scanning activities.
- Limit services’ exposure: Restrict the services running on both public and private network segments.
- Apply security patches regularly: Keep your operating systems and applications up-to-date with security patches to protect against known vulnerabilities.
- Implement network segmentation: Divide your network into segments to limit the access and impact of a potential intrusion.
- Regular network audits: Conduct regular security audits to identify and rectify potential vulnerabilities.
Notable Cyber Attacks Involving Port Scans
- Morris Worm (1988): One of the first worms distributed through the internet, used a port scanning technique to find IP addresses of vulnerable computers.
- Sasser Worm (2004): Targeted a vulnerability in Microsoft Windows and employed port scanning to propagate itself through IP addresses and port numbers of Windows PCs.
- Conficker Worm (2008): Used a sophisticated port scanning algorithm to quickly spread across millions of computers worldwide.
- Mirai Botnet (2016): Leveraged port scans to locate vulnerable IoT devices, later used to create massive Distributed Denial of Service (DDoS) attacks.
- BlueKeep RDP Exploit (2019): Attackers used port scans to identify systems susceptible to the BlueKeep vulnerability, which affected Remote Desktop Protocol in older Microsoft Windows versions.
Top Firewall Products to Prevent Port Scans
- Bitdefender - Known for excellent security features and a comprehensive firewall.
- Norton - Offers superior protection with intelligent firewall capabilities.
- McAfee - Provides robust firewall features to help keep your network secure.
- Kaspersky - Features an advanced firewall designed to block harmful scans.
- ZoneAlarm - Offers powerful firewall tools to block unwanted network entries.