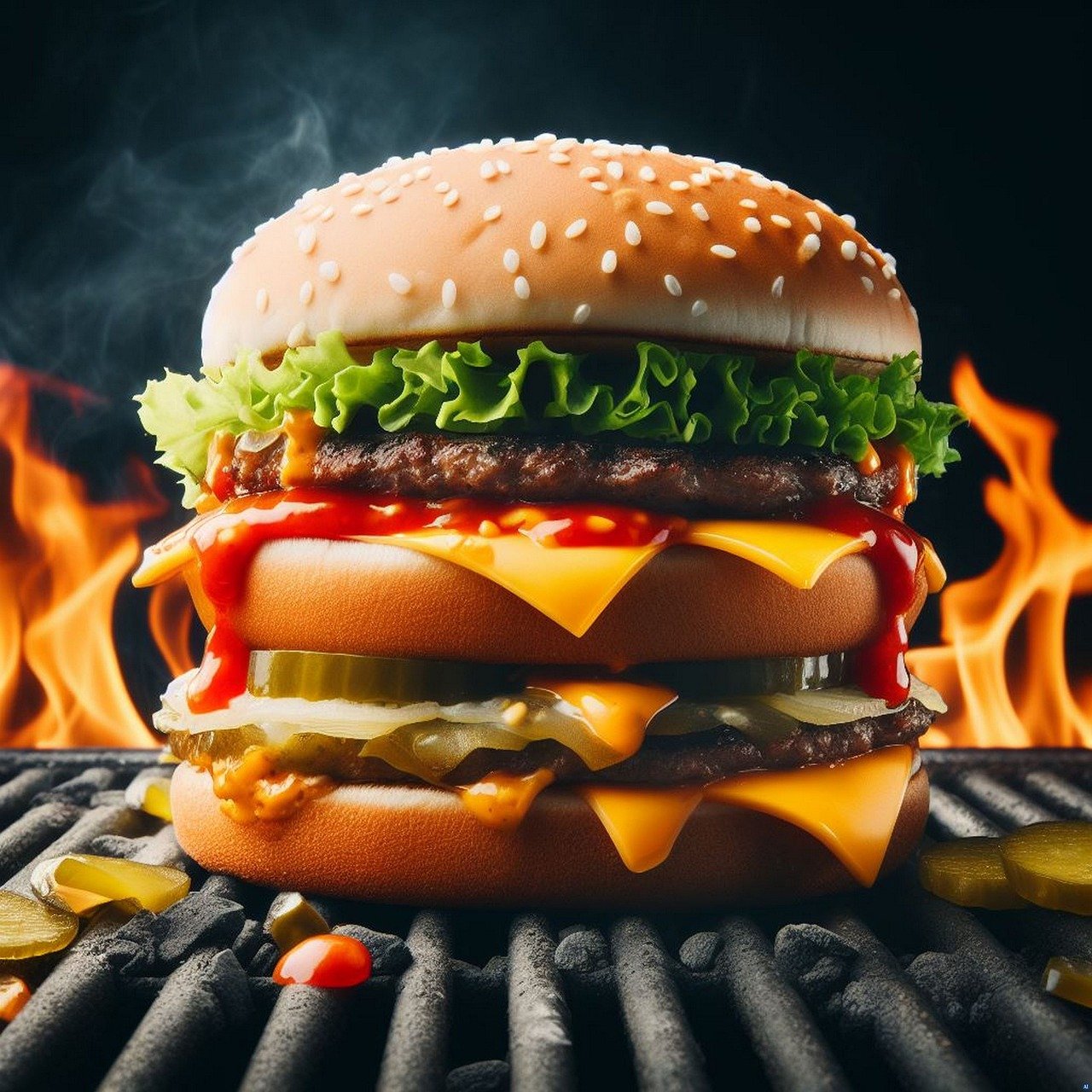
What's a MAC Address, How To Find MAC From IP in Python
A MAC (Media Access Control) address is a unique identifier assigned to network interfaces for communications on the physical network segment. Think of it as a serial number that uniquely identifies each device on a network.
MAC addresses are typically represented in hexadecimal format, with each octet (byte) separated by a colon (:) character. For example, the MAC address of a device connected to a network might be 00:1B:3E:4D:6F:80.
Whats the Relationship Between IP Address and MAC Address
In networking, the relationship between an IP address and a MAC address can be compared to a letter and its envelope. An IP address is like the postal address written on the envelope, guiding the data to the right destination across the broader network (internet). However, when data reaches a local network, the MAC address plays its role like a postal worker delivering the letter to the exact office or apartment within a large building. Thus, a single IP address can correspond to one or multiple MAC addresses in cases where a device has multiple network interfaces.
Using ARP to Find a MAC Address from an IP Address
The Address Resolution Protocol (ARP) is a vital networking utility used to map IP addresses to MAC addresses in a local network. ARP is essential in the data-link layer, facilitating the seamless exchange of data between devices in the same subnet.
If you know what is your IP or what's the IP of a device in a network, you can use the ARP utility to find the corresponding M A C address(Don't read it Max Address)
When you need to discover a device's MAC address on a local network, you can use the ARP command in the command-line interface. This command essentially queries the ARP cache, which contains mappings of IP addresses to MAC addresses. To use the ARP command, follow these steps:
- Open your command-line terminal.
- Enter the command
arp -a
to display the current ARP cache. - Look for the IP address you are interested in; the corresponding MAC address will be listed next to it.
By using ARP, you can easily find out which device possesses a certain IP address, thus enhancing network management and troubleshooting capabilities.
Python Code to Find MAC from IP Address
import subprocess
def get_mac_address(ip_address):
try:
pid = subprocess.Popen(["arp", "-a", ip_address], stdout=subprocess.PIPE)
s = pid.communicate()[0].decode('utf-8')
mac_address = None
for line in s.split('\n'):
if ip_address in line:
mac_address = line.split()[1]
break
if mac_address:
print(f"MAC address for {ip_address} is {mac_address}")
return mac_address
else:
print(f"MAC address for {ip_address} could not be found")
except Exception as e:
print(f"Exception: {e}")
How Bad Guys Can Use ARP to Map Your Network
Unfortunately, the ARP protocol is not very secure because it lacks authentication. This means that malicious actors, often referred to as "bad guys," can take advantage of ARP vulnerabilities to map out a network. One such method is ARP spoofing or ARP poisoning.
In an ARP spoofing attack, an attacker sends false ARP messages over a local network. This results in the linking of the attacker's MAC address with the IP address of a legitimate computer or server on the network. Once the attacker's MAC address is associated with an authentic IP address, they can start receiving any data meant for that IP address.
This kind of attack can lead to several problems, such as:
- Intercepting sensitive data: Attackers can capture important information like passwords, credit card numbers, etc., as these data packets traverse the network.
- Man-in-the-middle attacks: Attackers can alter the communication between two parties without their knowledge.
- Denial of service: Attackers can disrupt or completely block communications on the network.
To protect against ARP spoofing attacks, it's essential to use encrypted communication protocols (like HTTPS, SSH), keep the network secured with strong passwords, and employ network security tools that can detect and alert on suspicious ARP activity.
TCP/IP Socket Programming in C#